Data structures are fundamental in both technology and everyday experiences. They help organize and manage data efficiently, enabling engineers to create robust solutions. Let’s dive into some common data structures and their real-world applications.
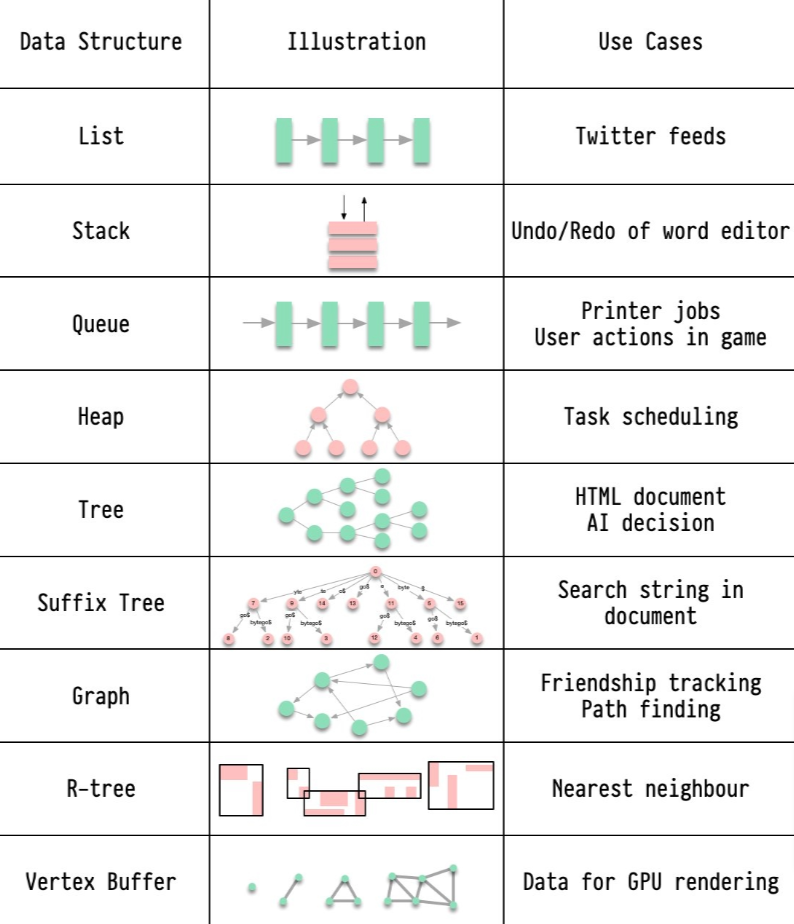
1. List
What It Is:
- A list is an ordered collection of elements that can be accessed by their position (index).
Daily Use:
- Twitter Feeds: Lists are used to store and manage the chronological sequence of tweets you see on your feed.
2. Stack
What It Is:
- A stack is a collection of elements with Last In, First Out (LIFO) access.
Daily Use:
- Undo/Redo in Word Editors: Stacks are used to keep track of actions for undoing and redoing operations in text editors.
3. Queue
What It Is:
- A queue is a collection of elements with First In, First Out (FIFO) access.
Daily Use:
- Printer Jobs: Queues manage print jobs, ensuring they are handled in the order they were received.
- In-Game User Actions: Queues send and process user actions in the sequence they occur in games.
4. Heap
What It Is:
- A heap is a specialized tree-based data structure that satisfies the heap property.
Daily Use:
- Task Scheduling: Heaps are used in priority queues for scheduling tasks based on priority.
5. Tree
What It Is:
- A tree is a hierarchical data structure consisting of nodes, with a single root and sub-nodes forming branches.
Daily Use:
- HTML Document: Trees organize the structure of HTML documents in web browsers.
- AI Decision Making: Trees are used in decision-making processes in artificial intelligence.
6. Suffix Tree
What It Is:
- A suffix tree is a compressed trie of all the suffixes of a given string.
Daily Use:
- Searching Strings in Documents: Suffix trees allow efficient substring searches within a document.
7. Graph
What It Is:
- A graph is a collection of nodes connected by edges.
Daily Use:
- Tracking Friendships: Graphs represent social networks, showing relationships and connections between individuals.
- Path Finding: Graphs are used in algorithms to find paths and routes, such as in GPS navigation.
8. R-Tree
What It Is:
- An R-tree is a tree data structure used for indexing multi-dimensional information.
Daily Use:
- Finding the Nearest Neighbor: R-trees are used in spatial databases to find the closest points or objects, such as in geographic information systems (GIS).
9. Vertex Buffer
What It Is:
- A vertex buffer is a memory buffer that stores vertex data used in rendering graphics.
Daily Use:
- Sending Data to GPU for Rendering: Vertex buffers send geometric data to the GPU for rendering 3D graphics in applications and games.
Conclusion
Data structures play a crucial role in our daily lives, both in the technology we use and the experiences we have. Engineers must understand these data structures and their practical applications to design effective and efficient solutions. By leveraging the right data structures, we can improve the performance and functionality of various systems and applications.